- This exercise is based on the material from Chapters 1-3.
- Review the code on pages 142-143.
- Watch applicable youtubes.
- Post your files to the student web server and put the corresponding URL in the Independent Exercise 1 drop box.
- Warning! WRITE ALL OF THE code FROM SCRATCH! Do not modify existing files from the book, your neighbor, codepen, your mother, or any other entity.
- Note color coding: keyword, blue: HTML, pink: CSS, green: JavaScript, black: general instructions)
Instructions
Using an html, linked css, and external js file...
- Create a
friend
object using literal syntax that includes these properties (no, the values do not need to be real, but they do need to be realistic):firstName
(assign a string literal)lastName
(assign a string literal)birthDate
(assign the date of May 5, 1992, usingnew Date()
)desiredAnnualSalary
(assign a reasonable annual salary, a numeric literal)educationLevel
(assign a numeric literal from 8-20 representing 8th grade through 8 years post high school)calculateSalary
(create a method that returns a variable namedlikelyAnnualSalary
calculated as thedesiredSalary
divided by 2 pluseducationLevel
multiplied by 100)
- Using JavaScript, write out the value of each of these properties to a paragraph element in the web page with id attributes of
id="p1"
throughid="p6"
. Include explanatory text so that each property value is identified. console.log
your friend'sbirthDate
property value to make sure you have created and converted it correctly
Hint: use thetoLocaleString()
method to convert salary values to include commas.- Create a function that converts a date to a
mm/dd/yyyy
format and use that function to format the date in the paragraph that writes out your friend's birth date. - Surround all of your code with an immediately invoked function expression.
- Style your html with a new, linked style sheet using responsive principles and best practices.
- Include a couple of basic media queries to make the page render well on a tablet and phone device.
- Include an appropriate meta viewport tag in the html.
- As always, follow best practices as outlined on the Homework Checklist.
Your final html file should look similar to the following in a browser: (Your text, data and styles will obviously differ. Also note the console.log
value.)
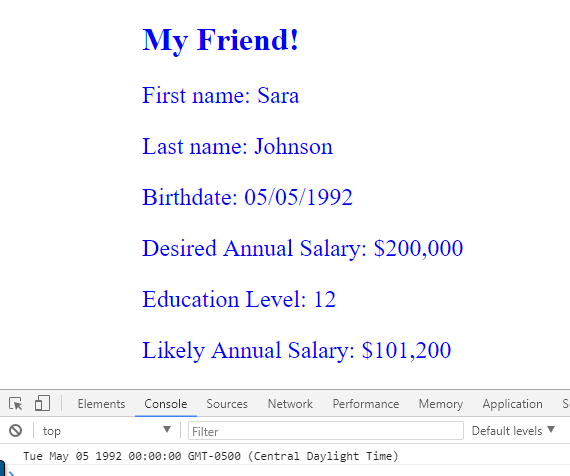